In this article, the scanf () function is considered in a general form without reference to a specific standard, therefore, data from any C99, C11, C ++ 11, C ++ 14 standards is included here. Perhaps in some standards the function works with differences from the material presented in the article.
Scanf C function - description
scanf () is a function located in the header file stdio.h (C) and cstdio (C ++), it is also called formatted data input into the program. scanf reads characters from the standard input stream (stdin) and converts them according to the format, and then writes them to the specified variables. Format - means that the data upon receipt is reduced to a certain form. Thus, the scanf C function is described:
scanf ("% format", & variable1 [, & variable2, [...]]),
where the variables are passed as addresses. The reason for this method of passing variables to a function is obvious: as a result of work, it returns a value indicating the presence of errors, so the only way to change the values ββof variables is to pass to the address. Also, thanks to this method, the function can process any type of data.
Some programmers, because of their analogy with other languages, call functions like scanf () or printf () procedures.
Scanf allows you to enter all the basic types of language: char, int, float, string, etc. In the case of variables of type string, there is no need to specify the address sign - β&β, since a variable of type string is an array, and its name is the address of the first element of the array in computer memory.
Data input format or control string
Let's start by looking at an example of using the scanf C function from the description.
#include <stdio.h> int main() { int x; while (scanf("%d", &x) == 1) printf("%d\n", x); return 0;
The input format consists of the following four parameters:% [*] [width] [modifiers] type. In this case, the β%β sign and type are required parameters. That is, the minimum form of the format is as follows: β% sβ, β% dβ and so on.
In general, the characters that make up the format string are divided into:
- format specifiers - everything that starts with the% character;
- delimiters or whitespace characters - they are considered space, tab (\ t), new line (\ n);
- characters other than whitespace.
The function may not be safe.
Use scanf_s () instead of scanf ().
(message from Visual Studio)
Type, or format specifiers, or conversion characters, or control characters
Description scanf C must contain at least a format specifier, which is indicated at the end of expressions starting with the β%β sign. It tells the program the type of data to be expected as you type, usually from the keyboard. A list of all format specifiers in the table below.
No. | A type | Value |
1 | % c | The program is waiting for a character to be entered. The variable for writing must be of char character type. |
2 | % d | The program expects to enter a decimal number of an integer type. The variable must be of type int. |
3 | % i | The program expects to enter a decimal number of an integer type. The variable must be of type int. |
4 | % e,% E | The program expects input of a floating point number (comma) in exponential form. The variable must be of type float. |
5 | % f | The program expects input of a floating point number (comma). The variable must be of type float. |
6 | % g,% G | The program expects input of a floating point number (comma). The variable must be of type float. |
7 | % a | The program expects input of a floating point number (comma). The variable must be of type float. |
8 | % o | The program expects to enter an octal number. The variable must be of type int. |
9 | % s | The program expects a string entry. A string is a set of any characters up to the first separator character encountered. The variable must be of type string. |
10 | % x,% X | The program expects to enter a hexadecimal number. The variable must be of type int. |
eleven | % p | The variable is waiting for a pointer to be entered. The variable must be of type pointer. |
12 | % n | Writes an integer value to the variable equal to the number of characters read to the current moment by the scanf function. |
thirteen | % u | The program reads an unsigned integer. The type of the variable must be an unsigned integer. |
14 | % b | The program expects to enter a binary number. The variable must be of type int. |
fifteen | % [] | Set of scanned characters. The program expects input of characters from a limited pool specified between square brackets. scanf will work as long as there are characters from the specified set on the input stream. |
16 | %% | The sign is "%". |
Characters in a format string
Asterisk symbol (*)
An asterisk (*) is a flag indicating that the assignment operation should be suppressed. An asterisk is placed immediately after the "%" sign. For instance,
scanf("%d%*c%d", &x, &y);
That is, if you enter the line β45-20β in the console, the program will do the following:
- The variable βxβ will be set to 45.
- The variable y will be set to 20.
- And the minus sign (dash) β-β will be ignored thanks to β% * cβ.
Width (or field width)
This is an integer between the β%β sign and the format specifier, which determines the maximum number of characters to read for the current read operation.
scanf("%20s", str);
There are several important points to keep in mind:
- scanf will terminate if it encounters a delimiter character, even if it does not count 20 characters.
- If more than 20 characters are input, only the first 20 of them will be written to the str variable.
Type modifiers (or precision)
These are special flags that modify the type of data expected to be entered. The flag is indicated to the left of the type specifier:
- L or l (small L) When using βlβ with qualifiers d, i, o, u, x, the flag tells the program that input of data of type long int is expected. When using βlβ with the e or f qualifier, the flag tells the program that it should expect to enter a double value. Using "L" tells the program that a value of type long double is expected. Using "l" with the qualifiers "c" and "s" tells the program that double-byte characters like wchar_t are expected. For example, "% lc", "% ls", "% l [asd]".
- h is a flag indicating the type of short.
- hh - indicates that the variable is a pointer to a value of type signed char or unsigned char. The flag can be used with qualifiers d, i, o, u, x, n.
- ll (two small L) - means that the variable is a pointer to a value of type signed long long int or unsigned long long int. The flag is used with qualifiers: d, i, o, u, x, n.
- j - indicates that the variable is a pointer to the type intmax_t or uintmax_t from the stdint.h header file. Used with qualifiers: d, i, o, u, x, n.
- z - means that the variable is a pointer to the type size_t, whose definition is in stddef.h. Used with qualifiers: d, i, o, u, x, n.
- t - indicates that the variable is a pointer to type ptrdiff_t. The definition for this type is in stddef.h. Used with qualifiers: d, i, o, u, x, n.
More clearly, the picture with modifiers can be represented in the form of a table. Such a description of scanf C for programmers will be clearer.
Other characters
Any characters that are encountered in the format will be discarded. It should be noted that the presence of whitespace or separation characters in the control line (new line, space, tab) can lead to different behavior of the function. In one version, scanf () will read without saving any number of delimiters until it encounters a character other than the delimiter, and in another version, spaces (only they) do not matter and the expression "% d +% d" is equivalent to "% d +% d ".
Examples
Consider a number of examples that allow us to reflect and more accurately understand the function.
scanf("%3s", str);
The above examples show how the expected number changes using different characters.
scanf C - description for beginners
This section will be useful for beginners. Often you need to have at hand not so much a complete description of scanf C as details of the function.
- The function is somewhat outdated. There are several different implementations in libraries of different versions. For example, the advanced scanf SC function, a description of which can be found on the microsoft website.
- The number of specifiers in the format should correspond to the number of arguments passed to the function.
- Elements of the input stream must be separated only by separation characters: space, tab, new line. Comma, semicolon, period, etc. - these characters are not separators for the scanf () function.
- If scanf encounters a delimiter, the input will stop. If there are more than one variable to read, then scanf will go on to read the next variable.
- The slightest discrepancy in the format of the input data leads to unpredictable results of the program. It is good if the program simply terminates with an error. But often the program continues to work and does it wrong.
- scanf ("% 20s ...", ...); If the input stream exceeds 20 characters, scanf will read the first 20 characters and either stop working or go on to read the next variable, if one is specified. In this case, the next scanf call will continue reading the input stream from the place where the previous scanf call stopped working. If a delimiter character is encountered when reading the first 20 characters, scanf will stop working or start reading the next variable, even if it does not count 20 characters for the first variable. In this case, all unread characters are attached to the next variable.
- If you start the set of scanned characters with a β^β, then scanf will read the data until it encounters a separator character or a character from the set. For example, "% [^ A-E1-5]" will read data from the stream until it encounters one of the English alphabet characters A to E in uppercase or one of the numbers 1 to 5.
- The scanf C function by description returns a number equal to the successful number of entries in the variables. If scanf writes 3 variables, the result of the successful operation of the function will be the return of the number 3. If scanf could not write any variables, the result will be 0. And finally, if scanf could not start working for any reason, the result will be EOF .
- If the scanf () function terminated incorrectly. For example, scanf ("% d", & x) - a number was expected, and characters entered the input. The next call to scanf () will start from the point in the input stream where the previous function call ended. To overcome this problem, you need to get rid of problematic characters. This can be done, for example, by calling scanf ("% * s"). That is, the function will read the character string and throw it away. In such a cunning way, you can continue to enter the necessary data.
- In some implementations of scanf (), the use of β-β is not allowed in the set of scanned characters.
- The qualifier β% cβ reads each character from the stream. That is, he also reads the delimiter character. To skip the delimiter character and continue reading the desired character, you can use β% 1sβ.
- When using the βcβ qualifier, it is permissible to use the width β% 10cβ, however, then an array of char elements must be passed as a variable to the scanf function.
- β% [Az]β means βall small letters of the English alphabetβ, and β% [za]β means just 3 characters: 'z', 'a', '-'. In other words, the symbol β-β means a range only if it is between two characters that are in the correct order. If β-β is at the end of an expression, at the beginning or in the wrong order of characters on either side of them, then it is just a hyphen, not a range.
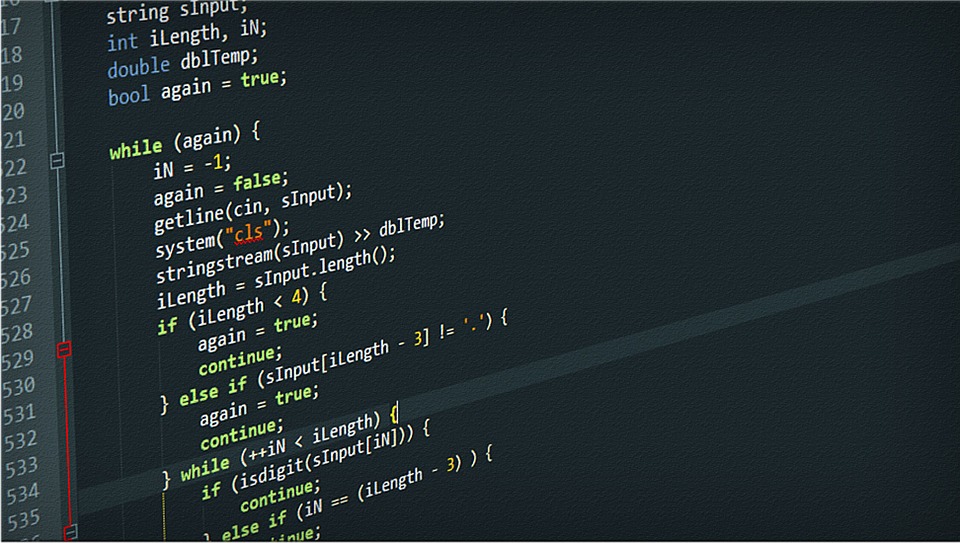
Conclusion
This completes the description of scanf C. This is a good convenient feature for working in small programs and using the procedural programming method. However, the main drawback is the number of unpredictable errors that can occur when using scanf. Therefore, the description of scanf C during programming is best kept in mind. In large professional projects, iostream streams are used, due to the fact that they have higher-level capabilities, they are better able to catch and process errors, as well as work with significant amounts of information. It should also be noted that the scanf C description in Russian is available on many network sources, as are examples of its use, due to the age of the function. Therefore, if necessary, you can always find the answer on thematic forums.