Python is a commonly used programming language, simple, beautiful, convenient. Working with him is a pleasure. Creating objects in Python (list, set, lines, files) is easy enough. The main thing is to understand the principle of programming and algorithmization.
List concept
A list is a grouped set enclosed in square brackets and is a very flexible data type. Elements are separated by commas. The list can be compared with the same array in Turbo Pascal or Delphi. In terms of physical location, such a grouped set is a list of addresses for its elements.
It is noteworthy that in Python a list can simultaneously contain various data types: integers and real numbers, strings, files, etc. In other programming languages, this is unacceptable.
Create lists. How to do it?
The most popular way to create grouped sets is through a generator. With this method, a specific expression is applied to each element of the list. The principle of operation of the generator can be compared with the FOR cycle.
You can turn a list into a string in Python and vice versa. To do this, set the list type to the selected string expression. As a result, the string turns into a grouped set of letters enclosed in quotation marks.
To create a more complex list of items, it is not recommended to use a list generator. Python suggests organizing a FOR loop. Some tasks allow you to specify a list manually, i.e., the user lists the necessary data in square brackets, separated by commas.
List Methods
In Python, a list has such tricks that make life easier for the user. They are called methods. Here is a list of some of the tricks used:
- List1.append (x) - a method that allows you to add any item to the end of the list.
- List1.extened (list2) - Adding a grouped set of list2 to the end of list1. Simply put, extend allows you to combine two lists.
- List1.insert (i, x) - insert any element X at position i. For example, if you insert a new object at the beginning of the list (and not at the end, as with the append method), then the addresses of all components will be updated taking into account the entered information.
- List1.remove (x) - removal of component X. If there are several X, then the first one from the list is cut. If the specified component does not appear in the list, the program throws a ValueError (x not in list) error.
- List1.pop ([i]) - cut and return the item at position i. If the user does not specify an index, then the method removes the last component. Square brackets are necessary in order to indicate the optionality of the parameter, i.e., the position can be skipped.

- List1.index (x, [start], [end]) - returns the cell address of the first element in the range from start to end. These parameters can be entered by the user at the invitation of the program. It is also necessary to clarify that indexation begins with 0. That is, the address of the first element is 0, the second is 1, etc. For example, there is a list X = [1.78487,5.575,7.364]. Then X [0] = 1.78487, X [1] = 5.575, X [2] = 7.364.
- List1.count (x) - returns the amount of the component whose value is equal to X. This method is useful when there are a lot of identical elements in the list and you need to count them.
- List1.sort ([key = function]) - organization of sorting the list. By default, Python performs this operation in ascending order. However, you can write a function for sorting and specify the parameters by which the components will be rearranged.
- List1.reverse () - flipping the list, that is, the first element becomes the last, the next takes the position of the second from the end, etc.
- List1.copy () - copy a list.
What you need to know when using methods? They do not overwrite the list and do not assign a new value to another variable.
Built-in Listing Features
Built-in functions make life easier for the user:
- Print - displays various information on the screen. In Python 2.7, after the specified function, you do not need to bracket the data; in Python 3, this is mandatory. For example, in Python 2.7, the function will look like this: print "Number of places", kolichestvo (where quotation marks indicate an explanation, and a comma separated variable). In Python 3, the organization of output is as follows: print ("Number of Seats", kolichestvo). You can display any type of data on the screen.
- Len (list1) - in Python, the length of the list determines the number of elements in the list.
- Min - finding the minimum component in the list, regardless of what type they are.
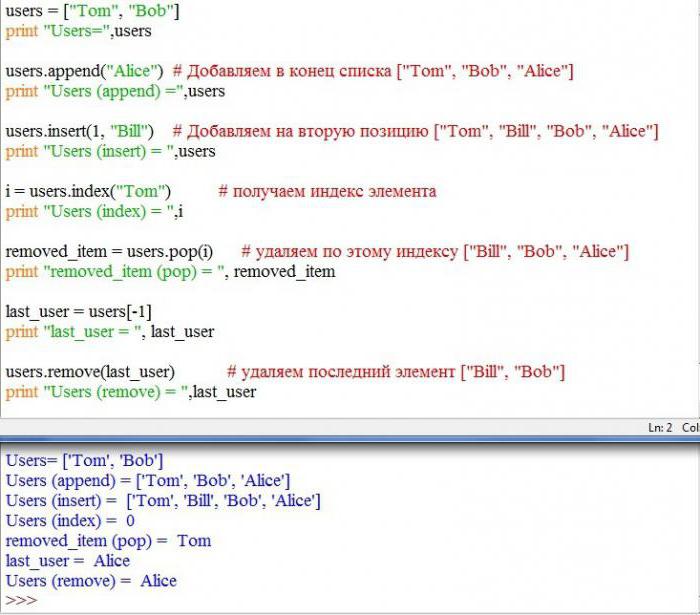
- Max - output of the maximum element in a grouped set.
- Sum - summation of numbers in the list, regardless of whether they are real or integer. If the grouped set has a string data type, then the program will throw a TypeError: unsupported operand type (s) for +: 'int' and 'str' error.
- Help - displays the help section for a specific data type. It is enough to write help (int) for integers, help (str) for a string data type, help (file) for files, and the program will provide help information in which you can find methods, objects and functions for the necessary section.
- Type - shows what type the given variable belongs to. It is formatted as follows: >>> text_str = "Hello" >>> type (text_str) >>> <type 'str'>
- In operator Of course, this is not a function, but with it you can check the occurrence of any element in the specified list.
Sort Lists
This programming language provides users with a lot of advantages. For example, in Python, sorting a list is organized simply. Unlike Turbo Pascal and Delphi, in which you sometimes need to write dozens of lines to align array elements in a certain order, in Python it is enough to create a function in 3-4 lines.
Tuples
Like strings, in Python a list can be an immutable object, in other words immutable. In this case, it is called a tuple, or tuple. The list requires more memory to complete the task. A tuple is enclosed in parentheses instead of square ones. Tuple output is allowed without parentheses at all. As said, a tuple is an immutable object. You cannot assign a value to a specific address. You can use the append method, which will add the necessary data to the end of the tuple. The following shows how to correctly add elements to a tuple.
If you use tuple as a function, it turns the string into a tuple. For example: tuple ("string") โ ("s", "t", "r", "i", "n", "g").
Lists and Files
Often, users do not know how to display a list of files in the Python language using a path. To do this, connect the OS module and use the listdir method. It should be noted that in Python 3 lists (in version 2.7 also) os.listdir (path) is required to enter the path to the directory in quotes instead of path. You can display the number of all objects in the folder.
Examples of tasks with a solution
1. Given a non-empty list. It is necessary to display the indexes of the elements of the list, if their values โโare in the specified interval. The minimum and maximum values โโof the interval are set by the user. The indices of the items found are written to the new list. Next, you need to display its contents and length.
Algorithm:
- Filling List1 randomly (i.e. randomly).
- Displays an invitation to the user to enter a minimum and maximum value for the interval.
- Organization of a cycle in which all elements of the initial list are enumerated, an index is searched for those components that are suitable for a given restriction (min <X <max).
- Adding items to the new List2 list.
- Display List2 and its length.
2. There is a non-empty list. You need to check if all the elements are unique, and make sure that there are no repetitions.
Algorithm:
- Create a list.
- Using the enumeration method, make sure that the given element and the subsequent one are different from each other.
- If a non-unique component appears, the message โNot uniqueโ is displayed.
- Exit the program.
Tasks for self-control
- Supplement to task 1. If an element with the desired value is found, it is added to the new list and removed from the old one. Hint: use while loop, len () function.
- A non-empty list is given. It is necessary to determine whether neighboring numbers are identical in sign (positive, negative). If such list items are present, you need to display them on the screen; if they are absent, do not display anything; if there are several pairs, display the first one.
- Create a list. The number of elements is set by the user. You need to generate a list in a random way and display elements located in even positions, starting from 0.

- Create a list. The number of elements is set by the user. You need to generate the list in a random way and display even elements, that is, divisible by 2. Hint: use the FOR loop. Attention: you need to iterate over numbers, not indexes.
- Create a list. The number of elements is set by the user. Generate a list in a random way. Display numbers that are larger than their previous neighbor.